SPI Lcd8 (8-bit interface) Library
The mikroPascal PRO for ARM provides a library for communication with Lcd (with HD44780 compliant controllers) in 8-bit mode via SPI interface.
For creating a custom set of Lcd characters use Lcd Custom Character Tool.

- When using this library with ARM family MCUs be aware of their voltage incompatibility with certain number of Lcd modules.
So, additional external power supply for these modules may be required. - Library uses the SPI module for communication. The user must initialize the appropriate SPI module before using the SPI Lcd8 Library.
- For MCUs with multiple SPI modules it is possible to initialize all of them and then switch by using the
SPI_Set_Active()
routine. See the SPI Library functions. - This Library is designed to work with the mikroElektronika's Serial Lcd/Glcd Adapter Board pinout, see schematic at the bottom of this page for details.
Library Dependency Tree
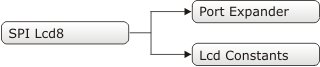
External dependencies of SPI Lcd Library
The implementation of SPI Lcd Library routines is based on Port Expander Library routines.
External dependencies are the same as Port Expander Library external dependencies.
Library Routines
SPI_Lcd8_Config
Prototype |
procedure SPI_Lcd8_Config(DeviceAddress : byte); |
---|---|
Description |
Initializes the Lcd module via SPI interface. |
Parameters |
|
Returns |
Nothing. |
Requires |
External dependencies are the same as Port Expander Library external dependencies.
|
Example |
Stellaris// Port Expander module connections var SPExpanderRST : sbit at GPIO_PORTA_DATA0_bit; SPExpanderCS : sbit at GPIO_PORTA_DATA1_bit; SPExpanderRST_Direction : sbit at GPIO_PORTA_DIR0_bit; SPExpanderCS_Direction : sbit at GPIO_PORTA_DIR1_bit; // End Port Expander module connections ... SPI1_Init(); // Initialize SPI module used with PortExpander SPI_Lcd8_Config(0); // intialize Lcd in 8bit mode via SPI MSP432// Port Expander module connections var SPExpanderRST : sbit at DIO_P6OUT.B0; SPExpanderCS : sbit at DIO_P6OUT.B1; SPExpanderRST_Direction : sbit at DIO_P6DIR.B0; SPExpanderCS_Direction : sbit at DIO_P6DIR.B1; // End Port Expander module connections ... // If Port Expander Library uses SPI module : SPI1_Init(); // Initialize SPI module used with PortExpander SPI_Lcd8_Config(0); STM32// Port Expander module connections var SPExpanderRST : sbit at GPIOB_ODR.B0; SPExpanderCS : sbit at GPIOB_ODR.B1; // End Port Expander module connections ... // Initialize SPI module used with PortExpander SPI1_Init_Advanced(_SPI_FPCLK_DIV4, _SPI_MASTER or _SPI_8_BIT or _SPI_CLK_IDLE_LOW or _SPI_FIRST_CLK_EDGE_TRANSITION or _SPI_MSB_FIRST or _SPI_SS_DISABLE or _SPI_SSM_ENABLE or _SPI_SSI_1, @_GPIO_MODULE_SPI1_PB345); SPI_Lcd8_Config(0); // intialize Lcd in 8bit mode via SPI CEC1x02// Port Expander module connections var SPExpanderRST : sbit at GPIO_OUTPUT_PIN_027_bit; SPExpanderCS : sbit at GPIO_OUTPUT_PIN_146_bit; // End Port Expander module connections ... // Initialize SPI module used with PortExpander SPI0_Init_Advanced(1000000,0,0); SPI_Lcd8_Config(0); |
Notes |
None. |
SPI_Lcd8_Out
Prototype |
procedure SPI_Lcd8_Out(row, column: byte; var text: string); |
---|---|
Description |
Prints text on Lcd starting from specified position. Both string variables and literals can be passed as a text. |
Parameters |
|
Returns |
Nothing. |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd8_Config routine. |
Example |
// Write text "Hello!" on Lcd starting from row 1, column 3: SPI_Lcd8_Out(1, 3, 'Hello!'); |
Notes |
None. |
SPI_Lcd8_Out_Cp
Prototype |
procedure SPI_Lcd8_Out_CP(var text: string); |
---|---|
Description |
Prints text on Lcd at current cursor position. Both string variables and literals can be passed as a text. |
Parameters |
|
Returns |
Nothing. |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd8_Config routine. |
Example |
// Write text "Here!" at current cursor position: SPI_Lcd8_Out_Cp('Here!'); |
Notes |
None. |
SPI_Lcd8_Chr
Prototype |
procedure SPI_Lcd8_Chr(row, column, out_char: byte); |
---|---|
Description |
Prints character on Lcd at specified position. Both variables and literals can be passed as character. |
Parameters |
|
Returns |
Nothing. |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd8_Config routine. |
Example |
// Write character "i" at row 2, column 3: SPI_Lcd8_Chr(2, 3, 'i'); |
Notes |
None. |
SPI_Lcd8_Chr_Cp
Prototype |
procedure SPI_Lcd8_Chr_CP(out_char: byte); |
---|---|
Description |
Prints character on Lcd at current cursor position. Both variables and literals can be passed as character. |
Parameters |
|
Returns |
Nothing. |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd8_Config routine. |
Example |
Print “e” at current cursor position: // Write character "e" at current cursor position: SPI_Lcd8_Chr_Cp('e'); |
Notes |
None. |
SPI_Lcd8_Cmd
Prototype |
procedure SPI_Lcd8_Cmd(out_char: byte); |
---|---|
Description |
Sends command to Lcd. |
Parameters |
|
Returns |
Nothing. |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd8_Config routine. |
Example |
// Clear Lcd display: SPI_Lcd8_Cmd(_LCD_CLEAR); |
Notes |
Predefined constants can be passed to the routine, see Available SPI Lcd8 Commands. |
Available SPI Lcd8 Commands
SPI Lcd8 Command | Purpose |
---|---|
_LCD_FIRST_ROW | Move cursor to the 1st row |
_LCD_SECOND_ROW | Move cursor to the 2nd row |
_LCD_THIRD_ROW | Move cursor to the 3rd row |
_LCD_FOURTH_ROW | Move cursor to the 4th row |
_LCD_CLEAR | Clear display |
_LCD_RETURN_HOME | Return cursor to home position, returns a shifted display to its original position. Display data RAM is unaffected. |
_LCD_CURSOR_OFF | Turn off cursor |
_LCD_UNDERLINE_ON | Underline cursor on |
_LCD_BLINK_CURSOR_ON | Blink cursor on |
_LCD_MOVE_CURSOR_LEFT | Move cursor left without changing display data RAM |
_LCD_MOVE_CURSOR_RIGHT | Move cursor right without changing display data RAM |
_LCD_TURN_ON | Turn Lcd display on |
_LCD_TURN_OFF | Turn Lcd display off |
_LCD_SHIFT_LEFT | Shift display left without changing display data RAM |
_LCD_SHIFT_RIGHT | Shift display right without changing display data RAM |