SPI Ethernet ENC24J600 Library
The ENC24J600
is a stand-alone Ethernet controller with an industry standard Serial Peripheral Interface (SPI).
It is designed to serve as an Ethernet network interface for any controller equipped with SPI.
The ENC24J600
meets all of the IEEE 802.3 specifications applicable to 10Base-T and 100Base-TX Ethernet.
It incorporates a number of packet filtering schemes to limit incoming packets. It also provides an internal, 16-bit wide DMA module for fast data throughput and hardware
assisted IP checksum calculations. Communication with the host controller is implemented via two interrupt pins and the SPI, with data rates of 10/100 Mb/s. Two dedicated pins are used for LED link and network activity indication.
This library is designed to simplify handling of the underlying hardware (ENC24J600
). It works with any ARM with
integrated SPI and more than 4 Kb ROM memory. 38 to 40 MHz clock is recommended to get from 8 to 10 Mhz SPI
clock, otherwise ARM should be clocked by ENC24J600
clock output due to its silicon bug in SPI hardware. If you try
lower ARM clock speed, there might be board hang or miss some requests.
SPI Ethernet ENC24J600 library supports:
- ENC424J600 and ENC624J600 modules.
- IPv4 protocol.
- ARP requests.
- ICMP echo requests.
- UDP requests.
- TCP requests (no stack, no packet reconstruction).
- ARP client with cache.
- DNS client.
- UDP client.
- DHCP client.
- packet fragmentation is NOT supported.

- Global library variable
SPI_Ethernet_24j600_userTimerSec
is used to keep track of time for all client implementations (ARP, DNS, UDP and DHCP). It is user responsibility to increment this variable each second in it's code if any of the clients is used. - For advanced users there is
__EthEnc24j600Private.mpas
unit in Uses folder of the compiler with description of all routines and global variables, relevant to the user, implemented in the SPI Ethernet ENC24J600 Library. - The appropriate hardware SPI module must be initialized before using any of the SPI Ethernet ENC24J600 library routines. Refer to SPI Library.
- For MCUs with multiple SPI modules it is possible to initialize them and then switch by using the
SPI_Set_Active()
routine.
Library Dependency Tree
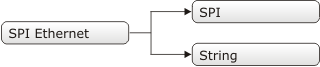
External dependencies of SPI Ethernet ENC24J600 Library
Stellaris
The following variables must be defined in all projects using SPI Ethernet ENC24J600 Library: | Description: | Examples : |
---|---|---|
var SPI_Ethernet_24j600_CS : sbit; sfr; external; |
ENC24J600 chip select pin. | var SPI_Ethernet_24j600_CS : sbit at GPIO_PORTA_DATA.B1; |
var SPI_Ethernet_24j600_CS_Direction : sbit; sfr; external; |
Direction of the ENC24J600 chip select pin. | var SPI_Ethernet_24j600_CS_Direction : sbit at GPIO_PORTA_DIR.B1; |
MSP432
The following variables must be defined in all projects using SPI Ethernet ENC24J600 Library: | Description: | Examples : |
---|---|---|
var SPI_Ethernet_24j600_CS : sbit; sfr; external; |
ENC24J600 chip select pin. | var SPI_Ethernet_24j600_CS : sbit at DIO_P6OUT.B1; |
var SPI_Ethernet_24j600_CS_Direction : sbit; sfr; external; |
Direction of the ENC24J600 chip select pin. | var SPI_Ethernet_24j600_CS_Direction : sbit at DIO_P6DIR.B1; |
STM32
The following variables must be defined in all projects using SPI Ethernet ENC24J600 Library: | Description: | Examples : |
---|---|---|
var SPI_Ethernet_24j600_CS : sbit; sfr; external; |
ENC24J600 chip select pin. | var SPI_Ethernet_24j600_CS : sbit at GPIOB_ODR.B1; |
CEC1x02
The following variables must be defined in all projects using SPI Ethernet ENC24J600 Library: | Description: | Examples : |
---|---|---|
var SPI_Ethernet_24j600_CS : sbit; sfr; external; |
ENC24J600 chip select pin. | var SPI_Ethernet_24j600_CS : sbit at GPIO_OUTPUT_PIN_146_bit; |
The following routines must be defined in all project using SPI Ethernet ENC24J600 Library: | Description: | Examples : |
---|---|---|
function SPI_Ethernet_24j600_UserTCP(var remoteHost : array[4] of byte, remotePort : word, localPort : word, reqLength : word) var flags: TEthj600PktFlags) : word; |
TCP request handler. | Refer to the library example at the bottom of this page for code implementation. |
function SPI_Ethernet_24j600_UserUDP(var remoteHost : array[4] of byte, remotePort : word, destPort : word, reqLength : word, var flags: TEthj600PktFlags) : word; |
UDP request handler. | Refer to the library example at the bottom of this page for code implementation. |
Library Routines
- SPI_Ethernet_24j600_Init
- SPI_Ethernet_24j600_Enable
- SPI_Ethernet_24j600_Disable
- SPI_Ethernet_24j600_doPacket
- SPI_Ethernet_24j600_putByte
- SPI_Ethernet_24j600_putBytes
- SPI_Ethernet_24j600_putString
- SPI_Ethernet_24j600_putConstString
- SPI_Ethernet_24j600_putConstBytes
- SPI_Ethernet_24j600_getByte
- SPI_Ethernet_24j600_getBytes
- SPI_Ethernet_24j600_UserTCP
- SPI_Ethernet_24j600_UserUDP
- SPI_Ethernet_24j600_setUserHandlers
- SPI_Ethernet_24j600_getIpAddress
- SPI_Ethernet_24j600_getGwIpAddress
- SPI_Ethernet_24j600_getDnsIpAddress
- SPI_Ethernet_24j600_getIpMask
- SPI_Ethernet_24j600_confNetwork
- SPI_Ethernet_24j600_arpResolve
- SPI_Ethernet_24j600_sendUDP
- SPI_Ethernet_24j600_dnsResolve
- SPI_Ethernet_24j600_initDHCP
- SPI_Ethernet_24j600_doDHCPLeaseTime
- SPI_Ethernet_24j600_renewDHCP
SPI_Ethernet_24j600_Init
Prototype |
procedure SPI_Ethernet_24j600_Init(mac: ^byte; ip: ^byte; fullDuplex: configuration); |
||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Description |
This is MAC module routine. It initializes ENC24J600 controller settings (parameters not mentioned here are set to default):
|
||||||||||||||
Parameters |
![]()
|
||||||||||||||
Returns |
Nothing. |
||||||||||||||
Requires |
External dependencies of the library from the top of the page must be defined before using this function.
|
||||||||||||||
Example |
Stellaris// mE ehternet NIC pinout var SPI_Ethernet_24j600_CS : sbit at GPIO_PORTA_DATA.B1 SPI_Ethernet_24j600_CS_Direction : sbit at GPIO_PORTA_DIR.B1; // end ethernet NIC definitions var myMacAddr : array[6] of byte; // my MAC address myIpAddr : array[4] of byte; // my IP addr ... myMacAddr[0] := 0x00; myMacAddr[1] := 0x14; myMacAddr[2] := 0xA5; myMacAddr[3] := 0x76; myMacAddr[4] := 0x19; myMacAddr[5] := 0x3F; myIpAddr[0] := 192; myIpAddr[1] := 168; myIpAddr[2] := 1; myIpAddr[3] := 60; SPI1_Init(); SPI_Ethernet_24j600_Init(myMacAddr, myIpAddr, SPI_Ethernet_24j600_MANUAL_NEGOTIATION and SPI_Ethernet_24j600_FULLDUPLEX and SPI_Ethernet_24j600_SPD100); MSP432// mE ehternet NIC pinout var SPI_Ethernet_24j600_CS : sbit at DIO_P6OUT.B1 SPI_Ethernet_24j600_CS_Direction : sbit at DIO_P6DIR.B1; // end ethernet NIC definitions var myMacAddr : array[6] of byte; // my MAC address myIpAddr : array[4] of byte; // my IP addr ... myMacAddr[0] := 0x00; myMacAddr[1] := 0x14; myMacAddr[2] := 0xA5; myMacAddr[3] := 0x76; myMacAddr[4] := 0x19; myMacAddr[5] := 0x3F; myIpAddr[0] := 192; myIpAddr[1] := 168; myIpAddr[2] := 1; myIpAddr[3] := 60; SPI1_Init(); SPI_Ethernet_24j600_Init(myMacAddr, myIpAddr, SPI_Ethernet_24j600_MANUAL_NEGOTIATION and SPI_Ethernet_24j600_FULLDUPLEX and SPI_Ethernet_24j600_SPD100); STM32// mE ehternet NIC pinout var SPI_Ethernet_24j600_CS : sbit at GPIOB_ODR.B1; // end ethernet NIC definitions var myMacAddr : array[6] of byte; // my MAC address myIpAddr : array[4] of byte; // my IP addr ... myMacAddr[0] := 0x00; myMacAddr[1] := 0x14; myMacAddr[2] := 0xA5; myMacAddr[3] := 0x76; myMacAddr[4] := 0x19; myMacAddr[5] := 0x3F; myIpAddr[0] := 192; myIpAddr[1] := 168; myIpAddr[2] := 1; myIpAddr[3] := 60; SPI3_Init_Advanced(_SPI_FPCLK_DIV16, _SPI_MASTER | _SPI_8_BIT | _SPI_CLK_IDLE_LOW | _SPI_FIRST_CLK_EDGE_TRANSITION | _SPI_MSB_FIRST | _SPI_SS_DISABLE | _SPI_SSM_ENABLE | _SPI_SSI_1, &_GPIO_MODULE_SPI3_PB345); SPI_Ethernet_24j600_Init(myMacAddr, myIpAddr, SPI_Ethernet_24j600_MANUAL_NEGOTIATION and SPI_Ethernet_24j600_FULLDUPLEX and SPI_Ethernet_24j600_SPD100); CEC1x02// mE ehternet NIC pinout var SPI_Ethernet_24j600_CS : sbit at GPIO_OUTPUT_PIN_146_bit; // end ethernet NIC definitions var myMacAddr : array[6] of byte; // my MAC address myIpAddr : array[4] of byte; // my IP addr ... myMacAddr[0] := 0x00; myMacAddr[1] := 0x14; myMacAddr[2] := 0xA5; myMacAddr[3] := 0x76; myMacAddr[4] := 0x19; myMacAddr[5] := 0x3F; myIpAddr[0] := 192; myIpAddr[1] := 168; myIpAddr[2] := 1; myIpAddr[3] := 60; SPI0_Init_Advanced(1000000,_SPI_MSB_FIRST,_SPI_SAMPLE_DATA_FALLING_EDGE OR _SPI_CLK_IDLE_LOW); SPI_Ethernet_24j600_Init(myMacAddr, myIpAddr, SPI_Ethernet_24j600_AUTO_NEGOTIATION); // init ethernet board |
||||||||||||||
Notes |
None. |
SPI_Ethernet_24j600_Enable
Prototype |
procedure SPI_Ethernet_24j600_Enable(enFlt : word); |
||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Description |
This is MAC module routine. This routine enables appropriate network traffic on the Advanced filtering available in the This routine will change receive filter configuration on-the-fly. It will not, in any way, mess with enabling/disabling receive/transmit logic or any other part of the |
||||||||||||||||||||||||||||||||||||
Parameters |
|
||||||||||||||||||||||||||||||||||||
Returns |
Nothing. |
||||||||||||||||||||||||||||||||||||
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
||||||||||||||||||||||||||||||||||||
Example |
SPI_Ethernet_24j600_Enable(_SPI_Ethernet_24j600_CRC or _SPI_Ethernet_24j600_UNICAST); // enable CRC checking and Unicast traffic |
||||||||||||||||||||||||||||||||||||
Notes |
Advanced filtering available in the This routine will change receive filter configuration on-the-fly. It will not, in any way, mess with enabling/disabling receive/transmit logic or any other part of the |
SPI_Ethernet_24j600_Disable
Prototype |
procedure SPI_Ethernet_24j600_Disable(disFlt : word); |
||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Description |
This is MAC module routine. This routine disables appropriate network traffic on the |
||||||||||||||||||||||||||||||||||||
Parameters |
|
||||||||||||||||||||||||||||||||||||
Returns |
Nothing. |
||||||||||||||||||||||||||||||||||||
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
||||||||||||||||||||||||||||||||||||
Example |
SPI_Ethernet_24j600_Disable(_SPI_Ethernet_24j600_CRC or _SPI_Ethernet_24j600_UNICAST); // disable CRC checking and Unicast traffic |
||||||||||||||||||||||||||||||||||||
Notes |
|
SPI_Ethernet_24j600_doPacket
Prototype |
function SPI_Ethernet_24j600_doPacket() : byte; |
---|---|
Description |
This is MAC module routine. It processes next received packet if such exists. Packets are processed in the following manner:
|
Parameters |
None. |
Returns |
|
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
while true do begin ... SPI_Ethernet_24j600_doPacket(); // process received packets ... end; |
Notes |
|
SPI_Ethernet_24j600_putByte
Prototype |
procedure SPI_Ethernet_24j600_putByte(v : byte); |
---|---|
Description |
This is MAC module routine. It stores one byte to address pointed by the current |
Parameters |
|
Returns |
Nothing. |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var data : byte; ... SPI_Ethernet_24j600_putByte(data); // put an byte into ENC24J600 buffer |
Notes |
None. |
SPI_Ethernet_24j600_putBytes
Prototype |
procedure SPI_Ethernet_24j600_putBytes(ptr : ^byte; n : word); |
---|---|
Description |
This is MAC module routine. It stores requested number of bytes into |
Parameters |
|
Returns |
Nothing. |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var buffer : array[17] of byte; ... buffer := 'mikroElektronika'; ... SPI_Ethernet_24j600_putBytes(buffer, 16); // put an RAM array into ENC24J600 buffer |
Notes |
None. |
SPI_Ethernet_24j600_putConstBytes
Prototype |
procedure SPI_Ethernet_24j600_putConstBytes(const ptr : ^byte; n : word); |
---|---|
Description |
This is MAC module routine. It stores requested number of const bytes into |
Parameters |
|
Returns |
Nothing. |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
const buffer : array[17] of byte; ... buffer := 'mikroElektronika'; ... SPI_Ethernet_24j600_putConstBytes(buffer, 16); // put a const array into ENC24J600 buffer |
Notes |
None. |
SPI_Ethernet_24j600_putString
Prototype |
function SPI_Ethernet_24j600_putString(ptr : ^byte) : word; |
---|---|
Description |
This is MAC module routine. It stores whole string (excluding null termination) into |
Parameters |
|
Returns |
Number of bytes written into |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var buffer : string[16]; ... buffer := 'mikroElektronika'; ... SPI_Ethernet_24j600_putString(buffer); // put a RAM string into ENC24J600 buffer |
Notes |
None. |
SPI_Ethernet_24j600_putConstString
Prototype |
function SPI_Ethernet_24j600_putConstString(const ptr : ^byte) : word; |
---|---|
Description |
This is MAC module routine. It stores whole const string (excluding null termination) into |
Parameters |
|
Returns |
Number of bytes written into |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
const buffer : string[16]; ... buffer := 'mikroElektronika'; ... SPI_Ethernet_24j600_putConstString(buffer); // put a const string into ENC24J600 buffer |
Notes |
None. |
SPI_Ethernet_24j600_getByte
Prototype |
function SPI_Ethernet_24j600_getByte() : byte; |
---|---|
Description |
This is MAC module routine. It fetches a byte from address pointed to by current |
Parameters |
None. |
Returns |
Byte read from |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var
buffer : byte;
...
buffer := SPI_Ethernet_24j600_getByte(); // read a byte from
|
Notes |
None. |
SPI_Ethernet_24j600_getBytes
Prototype |
procedure SPI_Ethernet_24j600_getBytes(ptr : ^byte; addr : word; n : word); |
---|---|
Description |
This is MAC module routine. It fetches equested number of bytes from |
Parameters |
|
Returns |
Nothing. |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var buffer: array[16] of byte; ... SPI_Ethernet_24j600_getBytes(buffer, 0x100, 16); // read 16 bytes, starting from address 0x100 |
Notes |
None. |
SPI_Ethernet_24j600_UserTCP
Prototype |
function SPI_Ethernet_24j600_UserTCP(var remoteHost : array[4] of byte; remotePort, localPort, reqLength : word; var flags: TEthj600PktFlags) : word; |
---|---|
Description |
This is TCP module routine. It is internally called by the library. The user accesses to the TCP request by using some of the SPI_Ethernet_24j600_get routines. The user puts data in the transmit buffer by using some of the SPI_Ethernet_24j600_put routines. The function must return the length in bytes of the TCP reply, or 0 if there is nothing to transmit. If there is no need to reply to the TCP requests, just define this function with return(0) as a single statement. |
Parameters |
|
Returns |
|
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
This function is internally called by the library and should not be called by the user's code. |
Notes |
The function source code is provided with appropriate example projects. The code should be adjusted by the user to achieve desired reply. |
SPI_Ethernet_24j600_UserUDP
Prototype |
function SPI_Ethernet_24j600_UserUDP(var remoteHost : array[4] of byte; remotePort, destPort, reqLength : word; var flags: TEthj600PktFlags) : word; |
---|---|
Description |
This is UDP module routine. It is internally called by the library. The user accesses to the UDP request by using some of the SPI_Ethernet_24j600_get routines. The user puts data in the transmit buffer by using some of the SPI_Ethernet_24j600_put routines. The function must return the length in bytes of the UDP reply, or 0 if nothing to transmit. If you don't need to reply to the UDP requests, just define this function with a return(0) as single statement. |
Parameters |
|
Returns |
|
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
This function is internally called by the library and should not be called by the user's code. |
Notes |
The function source code is provided with appropriate example projects. The code should be adjusted by the user to achieve desired reply. |
SPI_Ethernet_24j600_setUserHandlers
Prototype |
procedure SPI_Ethernet_24j600_setUserHandlers(TCPHandler : ^TSPI_Ethernet_24j600_UserTCP; UDPHandler : ^TSPI_Ethernet_24j600_UserUDP); |
---|---|
Description |
Sets pointers to User TCP and UDP handler function implementations, which are automatically called by SPI Ethernet ENC24J600 library. |
Parameters |
|
Returns |
Nothing. |
Requires |
SPI_Ethernet_24j600_UserTCP and SPI_Ethernet_24j600_UserUDP have to be previously defined. |
Example |
SPI_Ethernet_24j600_setUserHandlers(@SPI_Ethernet_24j600_UserTCP, @SPI_Ethernet_24j600_UserUDP); |
Notes |
Since all libraries are built for SSA, SSA restrictions regarding function pointers dictate that modules that use SPI_Ethernet_24j600_setUserHandlers must also be built for SSA. |
SPI_Ethernet_24j600_getIpAddress
Prototype |
function SPI_Ethernet_24j600_getIpAddress() : ^byte;
|
---|---|
Description |
This routine should be used when DHCP server is present on the network to fetch assigned IP address. |
Parameters |
None. |
Returns |
Pointer to the global variable holding IP address. |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var ipAddr : array[4] of byte; // user IP address buffer ... memcpy(ipAddr, SPI_Ethernet_24j600_getIpAddress(), 4); // fetch IP address |
Notes |
User should always copy the IP address from the RAM location returned by this routine into it's own IP address buffer. These locations should not be altered by the user in any case! |
SPI_Ethernet_24j600_getGwIpAddress
Prototype |
function SPI_Ethernet_24j600_getGwIpAddress() : ^byte;
|
---|---|
Description |
This routine should be used when DHCP server is present on the network to fetch assigned gateway IP address. |
Parameters |
None. |
Returns |
Pointer to the global variable holding gateway IP address. |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var gwIpAddr : array[4] of byte; // user gateway IP address buffer ... memcpy(gwIpAddr, SPI_Ethernet_24j600_getGwIpAddress(), 4); // fetch gateway IP address |
Notes |
User should always copy the IP address from the RAM location returned by this routine into it's own gateway IP address buffer. These locations should not be altered by the user in any case! |
SPI_Ethernet_24j600_getDnsIpAddress
Prototype |
function SPI_Ethernet_24j600_getDnsIpAddress() : ^byte;
|
---|---|
Description |
This routine should be used when DHCP server is present on the network to fetch assigned DNS IP address. |
Parameters |
None. |
Returns |
Pointer to the global variable holding DNS IP address. |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var dnsIpAddr : array[4] of byte; // user DNS IP address buffer ... memcpy(dnsIpAddr, SPI_Ethernet_24j600_getDnsIpAddress(), 4); // fetch DNS server address |
Notes |
User should always copy the IP address from the RAM location returned by this routine into it's own DNS IP address buffer. These locations should not be altered by the user in any case! |
SPI_Ethernet_24j600_getIpMask
Prototype |
function SPI_Ethernet_24j600_getIpMask() : ^byte;
|
---|---|
Description |
This routine should be used when DHCP server is present on the network to fetch assigned IP subnet mask. |
Parameters |
None. |
Returns |
Pointer to the global variable holding IP subnet mask. |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var IpMask : array[4] of byte; // user IP subnet mask buffer ... memcpy(IpMask, SPI_Ethernet_24j600_getIpMask(), 4); // fetch IP subnet mask |
Notes |
User should always copy the IP address from the RAM location returned by this routine into it's own IP subnet mask buffer. These locations should not be altered by the user in any case! |
SPI_Ethernet_24j600_confNetwork
Prototype |
procedure SPI_Ethernet_24j600_confNetwork(var ipMask, gwIpAddr, dnsIpAddr : array[4] of byte);
|
---|---|
Description |
Configures network parameters (IP subnet mask, gateway IP address, DNS IP address) when DHCP is not used. |
Parameters |
|
Returns |
Nothing. |
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var ipMask : array[4] of byte; // network mask (for example : 255.255.255.0) gwIpAddr : array[4] of byte; // gateway (router) IP address dnsIpAddr : array[4] of byte; // DNS server IP address ... gwIpAddr[0] := 192; gwIpAddr[1] := 168; gwIpAddr[2] := 20; gwIpAddr[3] := 6; dnsIpAddr[0] := 192; dnsIpAddr[1] := 168; dnsIpAddr[2] := 20; dnsIpAddr[3] := 100; ipMask[0] := 255; ipMask[1] := 255; ipMask[2] := 255; ipMask[3] := 0; ... SPI_Ethernet_24j600_confNetwork(ipMask, gwIpAddr, dnsIpAddr); // set network configuration parameters |
Notes |
The above mentioned network parameters should be set by this routine only if DHCP module is not used. Otherwise DHCP will override these settings. |
SPI_Ethernet_24j600_arpResolve
Prototype |
function SPI_Ethernet_24j600_arpResolve(var ip : array[4] of byte; tmax : byte) : ^byte; |
---|---|
Description |
This is ARP module routine. It sends an ARP request for given IP address and waits for ARP reply. If the requested IP address was resolved, an ARP cash entry is used for storing the configuration. ARP cash can store up to 3 entries. |
Parameters |
|
Returns |
|
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var IpAddr : array[4] of byte; // IP address ... IpAddr[0] := 192; IpAddr[0] := 168; IpAddr[0] := 1; IpAddr[0] := 1; ... SPI_Ethernet_24j600_arpResolve(IpAddr, 5); // get MAC address behind the above IP address, wait 5 secs for the response |
Notes |
The Ethernet services are not stopped while this routine waits for ARP reply. The incoming packets will be processed normaly during this time. |
SPI_Ethernet_24j600_sendUDP
Prototype |
function SPI_Ethernet_24j600_sendUDP(var destIP : array[4] of byte; sourcePort, destPort : word; pkt : ^byte; pktLen : word) : byte; |
---|---|
Description |
This is UDP module routine. It sends an UDP packet on the network. |
Parameters |
|
Returns |
|
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var IpAddr : array[4] of byte; // remote IP address ... IpAddr[0] := 192; IpAddr[0] := 168; IpAddr[0] := 1; IpAddr[0] := 1; ... SPI_Ethernet_24j600_sendUDP(IpAddr, 10001, 10001, 'Hello', 5); // send Hello message to the above IP address, from UDP port 10001 to UDP port 10001 |
Notes |
None. |
SPI_Ethernet_24j600_dnsResolve
Prototype |
function SPI_Ethernet_24j600_dnsResolve(var host : string; tmax : byte) : ^byte; |
---|---|
Description |
This is DNS module routine. It sends an DNS request for given host name and waits for DNS reply.
If the requested host name was resolved, it's IP address is stored in library global variable
and a pointer containing this address is returned by the routine. UDP port |
Parameters |
|
Returns |
|
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
var remoteHostIpAddr : array[4] of byte; // user host IP address buffer ... // SNTP server: // Zurich, Switzerland: Integrated Systems Lab, Swiss Fed. Inst. of Technology // 129.132.2.21: swisstime.ethz.ch // Service Area: Switzerland and Europe memcpy(remoteHostIpAddr, SPI_Ethernet_24j600_dnsResolve('swisstime.ethz.ch', 5), 4); |
Notes |
The Ethernet services are not stopped while this routine waits for DNS reply. The incoming packets will be processed normaly during this time. User should always copy the IP address from the RAM location returned by this routine into it's own resolved host IP address buffer. These locations should not be altered by the user in any case! |
SPI_Ethernet_24j600_initDHCP
Prototype |
function SPI_Ethernet_24j600_initDHCP(tmax : byte) : byte; |
---|---|
Description |
This is DHCP module routine. It sends an DHCP request for network parameters (IP, gateway, DNS addresses and IP subnet mask) and waits for DHCP reply. If the requested parameters were obtained successfully, their values are stored into the library global variables. These parameters can be fetched by using appropriate library IP get routines:
UDP port |
Parameters |
|
Returns |
|
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
... SPI_Ethernet_24j600_initDHCP(5); // get network configuration from DHCP server, wait 5 sec for the response ... |
Notes |
The Ethernet services are not stopped while this routine waits for DNS reply. The incoming packets will be processed normaly during this time. When DHCP module is used, global library variable |
SPI_Ethernet_24j600_doDHCPLeaseTime
Prototype |
function SPI_Ethernet_24j600_doDHCPLeaseTime() : byte; |
---|---|
Description |
This is DHCP module routine. It takes care of IP address lease time by decrementing the global lease time library counter. When this time expires, it's time to contact DHCP server and renew the lease. |
Parameters |
None. |
Returns |
|
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
while true do begin ... if (SPI_Ethernet_24j600_doDHCPLeaseTime() <> 0) then begin ... // it's time to renew the IP address lease end; end; |
Notes |
None. |
SPI_Ethernet_24j600_renewDHCP
Prototype |
function SPI_Ethernet_24j600_renewDHCP(tmax : byte) : byte; |
---|---|
Description |
This is DHCP module routine. It sends IP address lease time renewal request to DHCP server. |
Parameters |
|
Returns |
|
Requires |
Ethernet module has to be initialized. See SPI_Ethernet_24j600_Init. |
Example |
while true do begin ... if (SPI_Ethernet_24j600_doDHCPLeaseTime() <> 0) then begin SPI_Ethernet_24j600_renewDHCP(5); // it's time to renew the IP address lease, with 5 secs for a reply end; ... end; |
Notes |
None. |